Encryption/Dycription explained using .Net
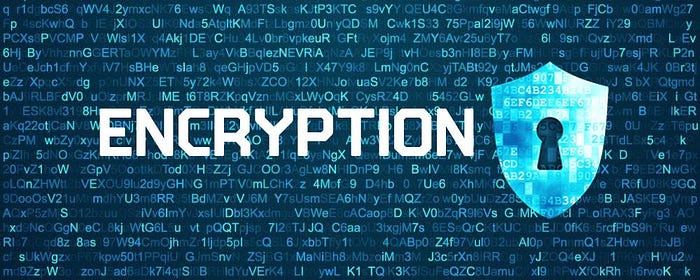
What is Encryption
Encryption is a process of converting information or data into a code to prevent unauthorized access. There are various encryption types, each with its own algorithms and methods. Here are some common encryption types:
1. Symmetric Key Encryption
- AES (Advanced Encryption Standard): Widely used symmetric encryption algorithm. It comes in different key sizes (128-bit, 192-bit, 256-bit) and is considered very secure.
- DES (Data Encryption Standard): An older symmetric key algorithm, now considered insecure for many applications due to its small key size.
- 3DES (Triple DES): An improvement over DES, applying the DES algorithm three times to each data block for increased security.
2. Asymmetric Key Encryption:
- RSA (Rivest-Shamir-Adleman): A widely used asymmetric encryption algorithm. It uses a pair of public and private keys for encryption and decryption.
- Elliptic Curve Cryptography (ECC): An asymmetric encryption algorithm based on the mathematics of elliptic curves. It provides strong security with shorter key lengths compared to RSA.
- Diffie-Hellman Key Exchange: Used to securely exchange cryptographic keys over an insecure channel. It’s often used in combination with other algorithms for secure communication.
3. Hash Functions:
- SHA-256 (Secure Hash Algorithm 256-bit): A commonly used hash function that produces a fixed-size output (256 bits).
- MD5 (Message Digest Algorithm 5): An older hash function, now considered insecure for cryptographic purposes due to vulnerabilities.
4. Hybrid Encryption:
- Combining both symmetric and asymmetric encryption for improved security and efficiency. Often used in secure communication protocols.
5. End-to-End Encryption:
- Ensures that data is encrypted on the sender’s system and can only be decrypted by the intended recipient, preventing interception or eavesdropping.
6. Quantum Encryption:
- A field of study that explores cryptographic methods resistant to attacks by quantum computers, which have the potential to break many traditional encryption algorithms.
Encryption plays a crucial role in securing data, communications, and transactions in various applications, including online banking, e-commerce, and communication platforms. The choice of encryption type depends on the specific requirements and security considerations of the application or system in use.
What is Decryption
On other hand Decryption in .NET involves the process of transforming encrypted data back into its original, readable form. The .NET Framework provides various cryptographic classes and libraries to perform decryption using symmetric or asymmetric encryption algorithms. Here’s a general outline of how decryption is typically done in .NET:
Symmetric Decryption (Using AES as an Example):
1. Create a Symmetric Algorithm Instance:
Choose a symmetric encryption algorithm such as AES (Advanced Encryption Standard).
using System.Security.Cryptography;
using (Aes aesAlg = Aes.Create())
{
// Set up the encryption algorithm parameters (key, IV, etc.)
}
2. Initialize the Algorithm Parameters:
Set up the necessary parameters, such as the encryption key and initialization vector (IV).
aesAlg.Key = keyBytes; // Replace keyBytes with your actual key
aesAlg.IV = ivBytes; // Replace ivBytes with your actual IV
3. Create a Decryptor:
Use the symmetric algorithm instance to create a decryptor.
ICryptoTransform decryptor = aesAlg.CreateDecryptor();
4. Decrypt the Data:
Apply the decryptor to the encrypted data.
byte[] decryptedBytes = decryptor.TransformFinalBlock(encryptedBytes, 0, encryptedBytes.Length);
Asymmetric Decryption (Using RSA as an Example):
1. Create an RSA Algorithm Instance:
Choose an asymmetric encryption algorithm such as RSA.
using System.Security.Cryptography;
using (RSA rsaAlg = RSA.Create())
{
// Set up the encryption algorithm parameters (key, padding, etc.)
}
2. Initialize the Algorithm Parameters:
Set up the necessary parameters, such as the private key.
rsaAlg.ImportRSAPrivateKey(privateKeyBytes, out _); // Replace privateKeyBytes with your actual private key
2. Decrypt the Data:
Use the RSA algorithm instance to decrypt the data.
byte[] decryptedBytes = rsaAlg.Decrypt(encryptedBytes, RSAEncryptionPadding.OaepSHA256);
Putting it all together (Using AES for Symmetric Decryption):
using System;
using System.Security.Cryptography;
using System.Text;
class Program
{
static void Main()
{
// Replace these with your actual key and IV
byte[] keyBytes = Encoding.UTF8.GetBytes("0123456789ABCDEF");
byte[] ivBytes = Encoding.UTF8.GetBytes("1234567890ABCDEF");
// Replace this with your actual encrypted data
byte[] encryptedBytes = Convert.FromBase64String("YOUR_BASE64_ENCODED_ENCRYPTED_DATA");
using (Aes aesAlg = Aes.Create())
{
aesAlg.Key = keyBytes;
aesAlg.IV = ivBytes;
ICryptoTransform decryptor = aesAlg.CreateDecryptor();
byte[] decryptedBytes = decryptor.TransformFinalBlock(encryptedBytes, 0, encryptedBytes.Length);
string decryptedText = Encoding.UTF8.GetString(decryptedBytes);
Console.WriteLine("Decrypted Text: " + decryptedText);
}
}
}
Remember to handle key management securely, and use proper error handling to handle exceptions that might occur during the decryption process. Always follow best practices for encryption and decryption to ensure the security of your application.
Conclusion
By understanding and applying encryption and decryption correctly, developers can build secure applications, protect sensitive information, and foster trust in an increasingly interconnected digital landscape. As technology evolves, staying informed about advancements like quantum encryption and adhering to best practices will remain vital for maintaining strong security measures.